Redis Data Structures: A Pythonic Interface for Distributed Data Structures
Introducing redis-data-structures - A Python library providing high-level, Redis-backed data structures with a clean, Pythonic interface.
As distributed systems become increasingly prevalent in modern software architectures, managing shared state and coordinating between multiple workers presents unique challenges. Today, I'm excited to introduce redis-data-structures, a Python library that provides high-level, Redis-backed data structures for building scalable and resilient applications.
The Problem Space
In distributed environments, we often need data structures that are:
- Thread-safe for concurrent access
- Persistent across process restarts
- Accessible from multiple workers/services
- Efficient for real-time operations
While Redis provides excellent primitives for building such structures, directly working with its commands can be verbose and error-prone. This is where redis-data-structures comes in. These challenge has led me down a fascinating path of system design that culminates in this library.
Let's unpack what makes this problem space particularly interesting:
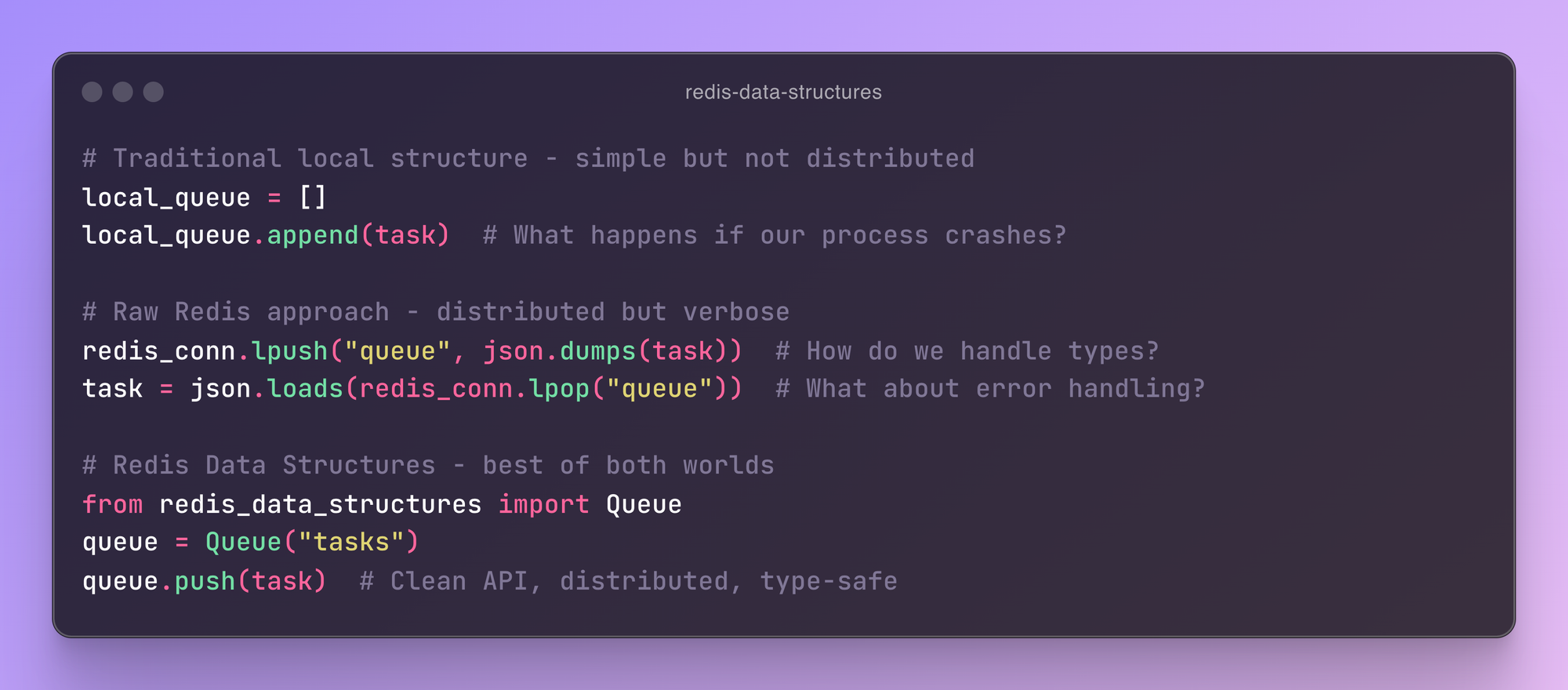
The library is built on three core engineering principles:
- Pythonic Abstraction: The API should feel natural to Python developers while preserving Redis's performance characteristics.
- Type Safety: Complex Python types should just work, without manual serialization gymnastics.
- Operational Resilience: Production systems need robust error handling and connection management.
Data Structures: A System Design Deep Dive
Each data structure is carefully engineered to maintain its theoretical time complexity while adding distributed capabilities:
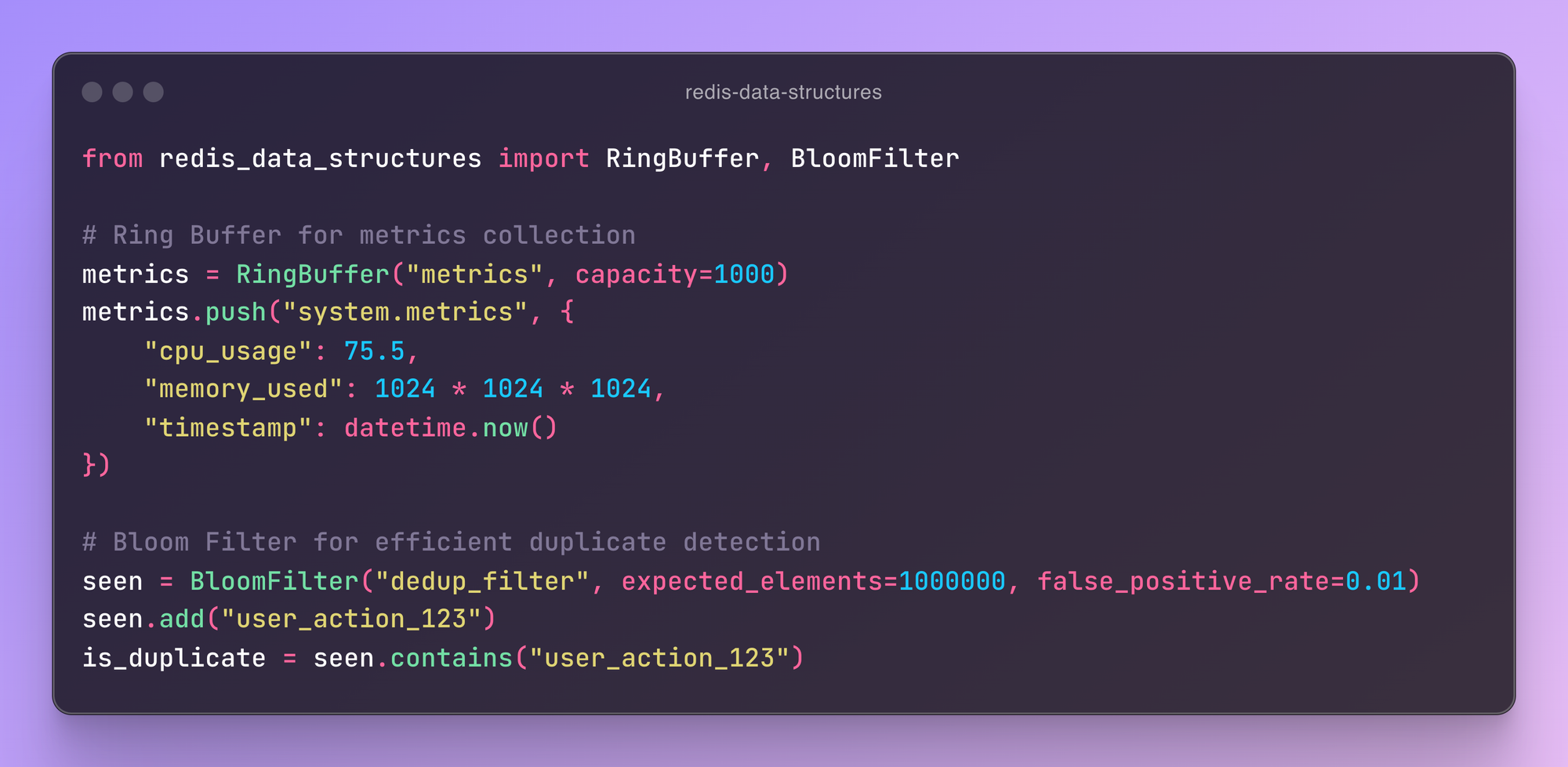
The RingBuffer implementation, for instance, uses Redis's LPOP
operation to maintain a fixed-size buffer with %O(1)% complexity - a beautiful example of mapping Redis primitives to higher-level data structures.
The library implements ten fundamental data structures, each optimized for specific use cases:
Structure | Description | Use Case |
---|---|---|
Queue | FIFO queue | Job processing, message passing |
Stack | LIFO stack | Undo systems, execution contexts |
Set | Unique collection | Membership testing, deduplication |
HashMap | Key-value store | Caching, metadata storage |
PriorityQueue | Priority-based queue | Task scheduling |
RingBuffer | Fixed-size circular buffer | Logs, metrics |
Graph | Graph with adjacency list | Relationships, networks |
Trie | Prefix tree | Autocomplete, spell checking |
BloomFilter | Probabilistic set | Membership testing |
Deque | Double-ended queue | Sliding windows |
Each structure maintains its expected time complexity while adding the benefits of persistence and distributed access.
Type System Engineering
One of the most technically interesting aspects of the library is its type preservation system. Rather than settling for simple string serialization, we've implemented a sophisticated type registry that maintains Python's rich type information:
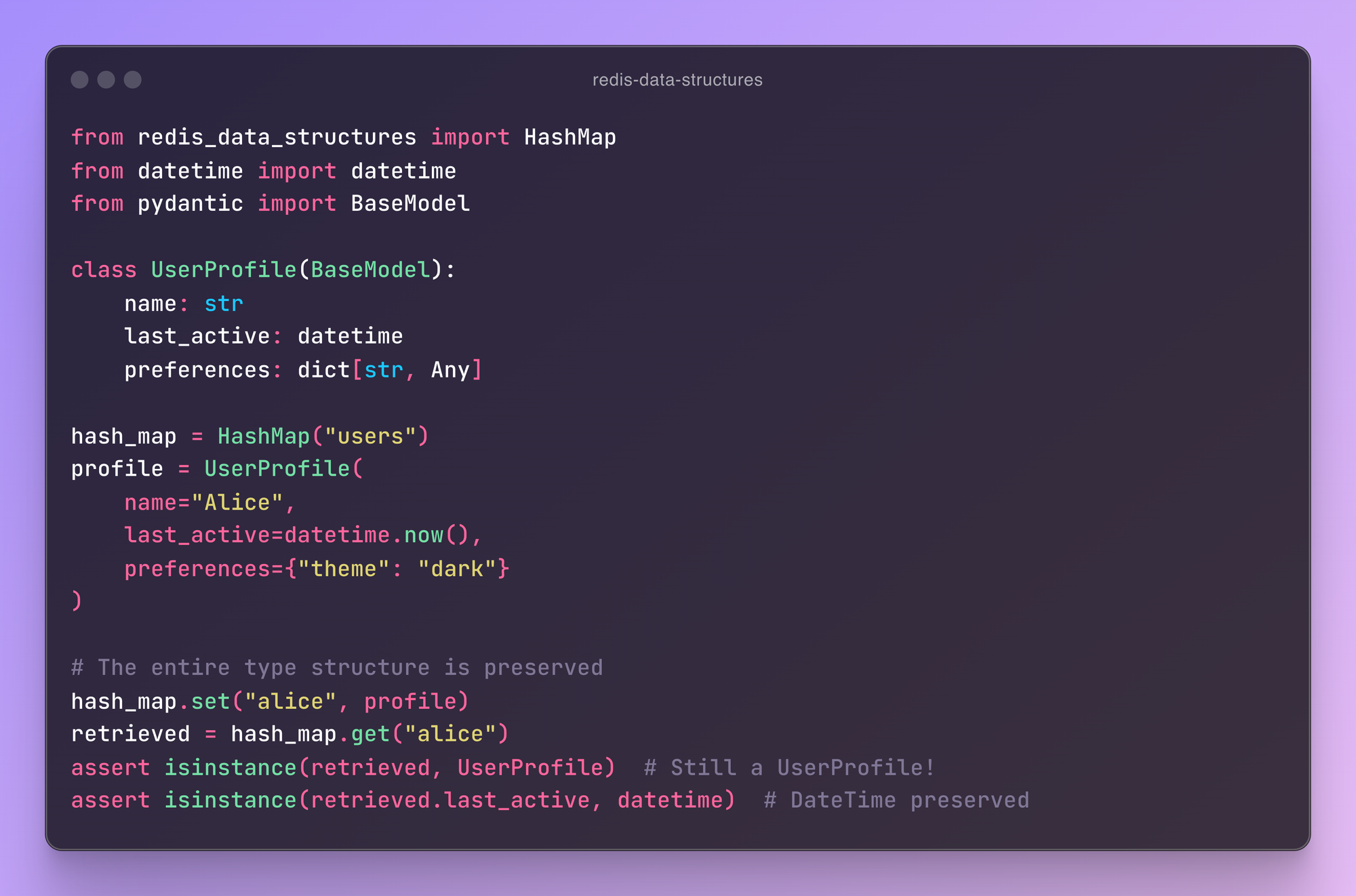
Connection Management
The connection management system is a testament to production engineering requirements. It implements the circuit breaker pattern to handle Redis failures gracefully:
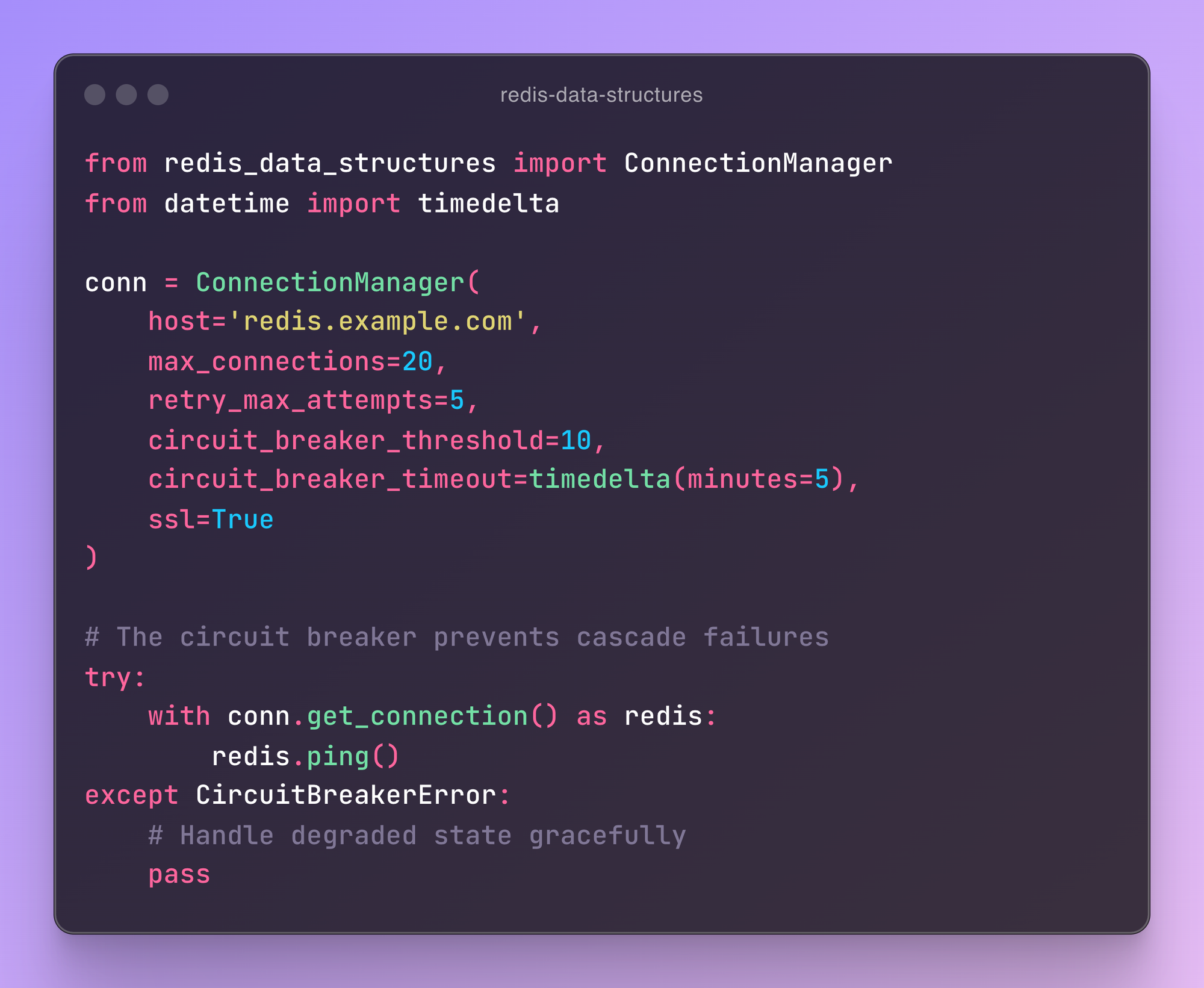
Real-World Engineering Application
Let's examine a real-world scenario - implementing a distributed rate limiter:
And another task scheduling with PriorityQueue:
These examples demonstrates how the library enables building sophisticated distributed systems with clean, maintainable code. See more examples here.
Getting Started
Installation is straightforward:
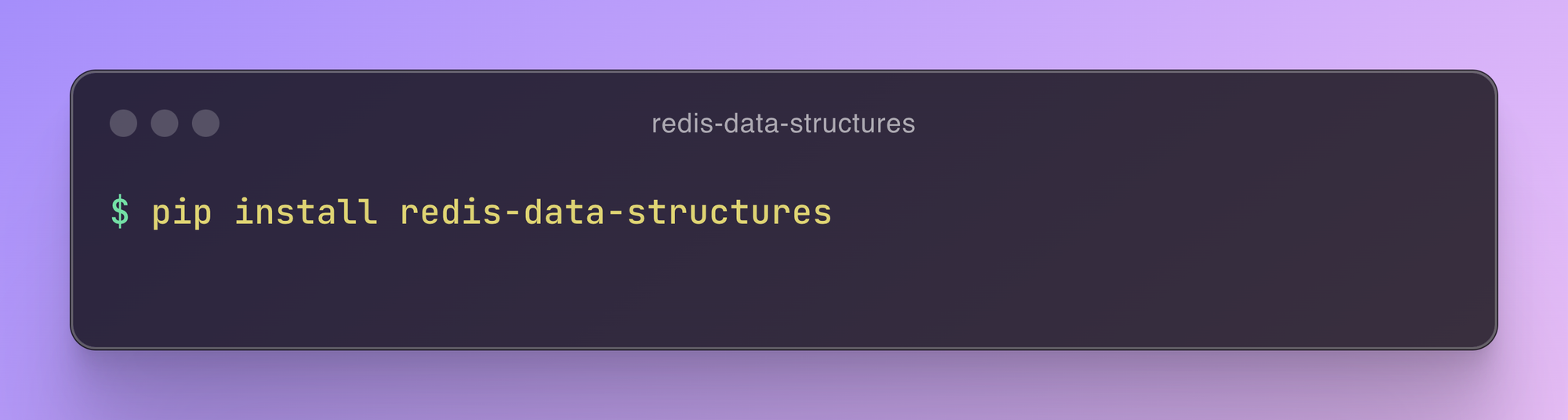
The library has been rigorously tested on Python 3.8+ and requires an active Redis instance. You can configure the connection settings either through environment variables for deployment flexibility or programmatically via the ConnectionManager
for fine-grained control.
Looking Ahead
The project is actively developed with exciting features on the roadmap:
- Async/await support for enhanced performance
- Additional data structure implementations
- Enhanced monitoring and debugging capabilities
- Extended type system support