Concurrency Programming in Python: Thread vs. Process
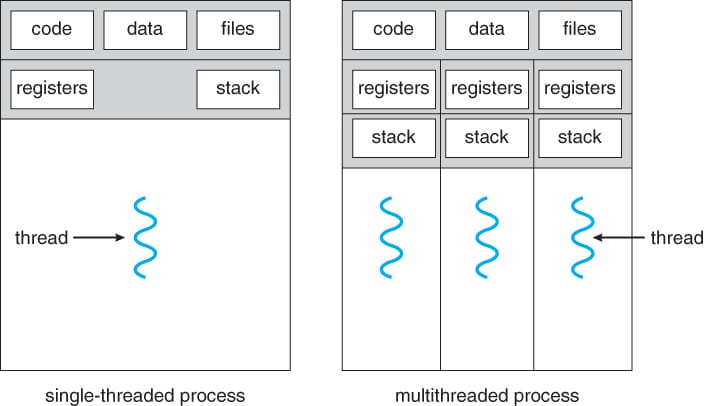
Concurrency is a vital aspect of modern programming, allowing applications to perform multiple tasks simultaneously, thus improving efficiency and responsiveness. In Python, developers often face the choice between using threads or processes to achieve concurrency. This blog explores the differences between these two concurrency models, how they interact with Python's Global Interpreter Lock (GIL), and best practices for handling I/O-bound and CPU-bound tasks.
Understanding Threads and Processes
Threads
A thread is the smallest unit of execution within a process. Threads within the same process share memory space, allowing for efficient data sharing but requiring careful synchronization to avoid race conditions.
Advantages:
- Lightweight and faster to create compared to processes.
- Shared memory space facilitates easy data sharing.
Disadvantages:
- Requires synchronization mechanisms to prevent race conditions.
- In CPython, threads are limited by the GIL, affecting their performance for CPU-bound tasks.
Processes
A process is an independent program in execution, with its own memory space. Processes are more isolated than threads, making inter-process communication (IPC) more complex but safer in terms of memory management.
Advantages:
- Each process has its own memory space, reducing the risk of memory corruption.
- Can achieve true parallelism in Python, bypassing the GIL.
Disadvantages:
- More resource-intensive to create and manage compared to threads.
- IPC is more complex and slower.
The Global Interpreter Lock (GIL)
The Global Interpreter Lock (GIL) is a mutex that protects access to Python objects, preventing multiple native threads from executing Python bytecodes at once. This lock is necessary because CPython's memory management is not thread-safe. The GIL ensures that only one thread executes Python bytecode at a time, which simplifies the implementation of CPython and avoids race conditions, but it also has significant implications for multi-threaded programs.
Why the GIL Exists:
- Memory Management: CPython uses reference counting for memory management. Without the GIL, the reference count updates could be corrupted by concurrent threads.
- Ease of Implementation: The GIL simplifies the CPython implementation, making it easier to integrate C extensions and manage memory.
Implications of the GIL:
- I/O-Bound Tasks: The GIL has minimal impact on I/O-bound tasks because it is released during I/O operations, allowing other threads to run.
- CPU-Bound Tasks: The GIL becomes a bottleneck, preventing threads from running in parallel and limiting the performance of CPU-bound tasks.
Handling I/O-Bound and CPU-Bound Tasks
I/O-Bound Tasks
I/O-bound tasks are operations where the system spends more time waiting for input/output operations to complete than performing computations. These tasks are often limited by the speed of external systems like disk drives, network connections, or other peripheral devices.
Characteristics of I/O-Bound Tasks:
- Waiting for External Resources: These tasks often involve waiting for data to be read from or written to a disk, network communication, or user input.
- Low CPU Utilization: The CPU is often idle, waiting for I/O operations to complete.
- Examples: Reading/writing files, database queries, network requests, user interactions.
Handling I/O-Bound Tasks:
- Multi-threading: Effective because while one thread waits for I/O, others can proceed with their work.
- Asynchronous Programming: Using non-blocking I/O operations (e.g., Python's
asyncio
, JavaScript'sasync/await
) to handle many I/O-bound tasks concurrently within a single thread.
CPU-Bound Tasks
CPU-bound tasks are operations where the system spends most of its time performing computations rather than waiting for I/O operations. These tasks are limited by the processing power of the CPU.
Characteristics of CPU-Bound Tasks:
- Intensive Computations: These tasks require significant CPU processing power.
- High CPU Utilization: The CPU is actively engaged in executing instructions.
- Examples: Mathematical computations, data processing, image rendering, machine learning model training.
Handling CPU-Bound Tasks:
- Multi-processing: Effective because it allows leveraging multiple CPU cores by creating separate processes (e.g., Python's
multiprocessing
module). - Compiled Languages: Using languages or extensions that allow direct machine code execution (e.g., C/C++, Cython for Python).
Achieving True Concurrency in Python
To achieve true concurrency in Python, you should determine whether your task is I/O-bound or CPU-bound and choose threads or processes accordingly. Here are two examples of different tasks implemented in different ways:
By understanding the nature of your tasks and leveraging the appropriate concurrency model, you can effectively improve the performance and responsiveness of your Python applications.